内部结构
通过查看源码可以知道,HashMap内部的基本结构是数组+链表的形式。
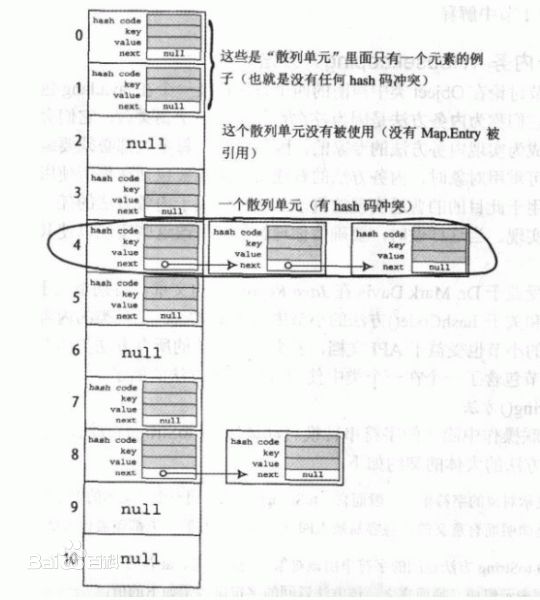
put()方法
通过源码得知调用put方法,最终会调用putVal方法
get()方法
|
|
总结
- 扩容时机:当哈希表中的结点数超出了加载因子与当前容量的乘积时
- HashMap中元素的索引并不恒定不变,当进行扩容时,索引可能会发生偏移,偏移量为2的n次方
学习经验交流
通过查看源码可以知道,HashMap内部的基本结构是数组+链表的形式。
通过源码得知调用put方法,最终会调用putVal方法
|
|
- 扩容时机:当哈希表中的结点数超出了加载因子与当前容量的乘积时
- HashMap中元素的索引并不恒定不变,当进行扩容时,索引可能会发生偏移,偏移量为2的n次方